Tutorial 10 - Walk-through ASP Examples
Contents
Web Form Examples
See the TeeChartForNET Virtual Share installed with TeeChart for a working example of WebForm and other ASP.NET examples written in C#.NET and VB.NET.
Web Form Examples
How to create a dynamic WebChart
private void Page_Load(object sender, System.EventArgs e)
{
string chartName=Request.QueryString["Chart"];
if (Session[chartName]!=null)
{
System.IO.MemoryStream chartStream = new System.IO.MemoryStream();
chartStream=((System.IO.MemoryStream)Session[chartName];
Response.OutputStream.Write(chartStream.ToArray(),0,(int)chartStream.Length);
chartStream.Close();
Session.Remove(chartName);
}
}
private void Page_Load(object sender, System.EventArgs e)Executing this code and moving the mouse over the bubbles will show you the values of the series marks, in this case, the YValues.
{
//Let's work with the Chart object for convenience
Steema.TeeChart.Chart Chart1 = WebChart1.Chart;
//Add in a series and fill it
Chart1.Aspect.View3D = false;
Steema.TeeChart.Styles.Bubble bubble1 = new Steema.TeeChart.Styles.Bubble(Chart1);
bubble1.FillSampleValues();
//Add a SeriesToolTip to the Chart
Steema.TeeChart.Tools.SeriesHotspot seriesHotSpot1 = new Steema.TeeChart.Tools.SeriesHotspot(Chart1);
//Steema.TeeChart.Styles.MapAction.Mark is the default value
seriesHotSpot1.MapAction = Steema.TeeChart.Styles.MapAction.Mark;
}
private void Page_Load(object sender, System.EventArgs e)
{
//Let's work with the Chart object for convenience
Steema.TeeChart.Chart Chart1 = WebChart1.Chart;
//Add in a series and fill it
Chart1.Aspect.View3D = false;
Steema.TeeChart.Styles.Bubble bubble1 = new Steema.TeeChart.Styles.Bubble(Chart1);
bubble1.FillSampleValues();
//Add a SeriesToolTip to the Chart
Steema.TeeChart.Tools.SeriesHotspot seriesHotSpot1 = new Steema.TeeChart.Tools.SeriesHotspot(Chart1);
//Steema.TeeChart.Styles.MapAction.Mark is the default value
seriesHotSpot1.MapAction = Steema.TeeChart.Styles.MapAction.Mark;
//Add a ZoomTool to the Chart
Steema.TeeChart.Tools.ZoomTool zoomTool1 = new Steema.TeeChart.Tools.ZoomTool(Chart1);
// *************** Code for zoom support ***************
//check whether zoom request is being sent
CheckZoom(WebChart1);
}
private void CheckZoom(WebChart wChart)
{
ArrayList zoomedState=(ArrayList)Session[wChart.ID+"Zoomed"];
zoomedState=((Steema.TeeChart.Tools.ZoomTool)wChart.Chart.Tools[0]).SetCurrentZoom(Request,zoomedState);
if (zoomedState==null)
Session.Remove(wChart.ID+"Zoomed");
else
Session.Add(wChart.ID+"Zoomed",zoomedState);
}
Within the Page_Load event:
seriesHotSpot1.MapAction = Steema.TeeChart.Styles.MapAction.URL;And the GetHTMLMap method:
seriesHotSpot1.GetHTMLMap += new Steema.TeeChart.Tools.SeriesHotspotEventHandler(seriesHotSpot1_GetHTMLMap);
private void seriesHotSpot1_GetHTMLMap(Steema.TeeChart.Tools.SeriesHotspot sender, Steema.TeeChart.Tools.SeriesHotspotEventArgs e)
{
e.PointPolygon.Title = "Go to the Steema web";
e.PointPolygon.HREF = "http://www.steema.com";
e.PointPolygon.Attributes = "target='_blank'";
}
Within the Page_Load event:
seriesHotSpot1.MapAction = Steema.TeeChart.Styles.MapAction.Script;
seriesHotSpot1.HelperScript = Steema.TeeChart.Tools.HotspotHelperScripts.Annotation;
The second line here ensures that the relevant JavaScript is added to the client browser.
And the GetHTMLMap method:
private void seriesHotSpot1_GetHTMLMap(Steema.TeeChart.Tools.SeriesHotspot sender, Steema.TeeChart.Tools.SeriesHotspotEventArgs e)
{
e.PointPolygon.Attributes=String.Format(Steema.TeeChart.Texts.HelperScriptAnnotation, "<IMG SRC=Images/myimage.jpg>");
}
To customize the behavior even further would simply mean designing your own JavaScript routines, adding them to the client browser and then calling them by adding them and their parameters to e.PointPolygon.Attributes.

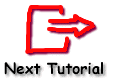