Tutorial 11 - Zoom and Scroll
Zoom and Scroll are useful aids for focusing on specific data in a densely populated Chart. Contents
How to Zoom and Scroll using the Mouse Zoom
Scroll
How to Zoom and Scroll by code
Zoom
Animated Zoom
Zoom events
Scroll
How to Zoom and Scroll using the Mouse
Zoom To zoom in on a Chart, press the left mousebutton at the top left hand corner of the area you wish to zoom in on and maintaining the mousebutton pressed, drag out the rectangle to the bottom righthand corner of the zoom area. Release the mousebutton and the Chart will redraw the area selected.
To undo the zoom, press the left mousebutton anywhere on the Chart area and drag up and left with the mousebutton depressed. Release the button and the Chart will redraw to the originally defined Chart area.
Scroll
To scroll a Chart across, press the right mousebutton and maintaining the mousebutton pressed, drag the mouse in the direction you wish to scroll the Chart. When you release the mousebutton the Chart will remain at the new location.
To undo the scroll, press the left mousebutton anywhere on the Chart area and drag up and left with the mousebutton depressed. Release the button and the Chart will redraw to the originally defined Chart area.
How to Zoom and Scroll by code
Zoom Zoom is enabled by default. Use the Zoom.Allow property to disable zoom. See the Zoom Class for a full list of properties and methods associated with Zoom. To define a rectangular area over which to Zoom use the ZoomRect method.
ExampleThe ZoomRect co-ordinates are defined in screen pixels where 0,0 is the top left hand point of the Chart Panel.
[C#]
tChart1.Zoom.ZoomRect(new Rectangle(100,100,120,120));
[VB.Net]
TChart1.Zoom.ZoomRect(New Rectangle(100, 100, 120, 120))
The following code will zoom in on an area between the 2nd and 5th x-axis points, setting the y-axis to the scale of the maximum and minimum points of the entire Chart:
[C#]
int x = points1.CalcXPos(2);
int y = tChart1.Axes.Left.CalcYPosValue(tChart1.Axes.Left.MaxYValue);
int height = tChart1.Axes.Left.CalcYPosValue(tChart1.Axes.Left.MinYValue) - tChart1.Axes.Left.CalcYPosValue(tChart1.Axes.Left.MaxYValue);
int width = points1.CalcXPos(5) - x;
Rectangle r = new Rectangle(x,y,width,height);
tChart1.Zoom.ZoomRect(r);
[VB.Net]
Dim X As Integer = Points1.CalcXPos(2)
Dim Y As Integer = TChart1.Axes.Left.CalcYPosValue(TChart1.Axes.Left.MaxYValue)
Dim Height As Integer = TChart1.Axes.Left.CalcYPosValue(TChart1.Axes.Left.MinYValue) - TChart1.Axes.Left.CalcYPosValue(TChart1.Axes.Left.MaxYValue)
Dim Width As Integer = Points1.CalcXPos(5) - X
Dim R As New Rectangle(X, Y, Width, Height)
TChart1.Zoom.ZoomRect(R)
Use 'Undo' to Zoom back out.
TChart1.Zoom.Undo
Animated Zoom
Animated Zoom offers stepped zooming. Instead of jumping from 'zoomed out' to 'zoomed in' in one step, you may set Animated to enabled and define staggered steps for the zoom. Once Animated is enabled you may zoom manually with the Mouse or by code.
Example
[C#]
int x = points1.CalcXPos(2);
int y = tChart1.Axes.Left.CalcYPosValue(tChart1.Axes.Left.MaxYValue);
int height = tChart1.Axes.Left.CalcYPosValue(tChart1.Axes.Left.MinYValue) - tChart1.Axes.Left.CalcYPosValue(tChart1.Axes.Left.MaxYValue);
int width = points1.CalcXPos(5) - x;
Rectangle r = new Rectangle(x,y,width,height);
tChart1.Zoom.Animated = true;
tChart1.Zoom.AnimatedSteps = 100;
tChart1.Zoom.ZoomRect(r);
[VB.Net]
Dim X As Integer = Points1.CalcXPos(2)
Dim Y As Integer = TChart1.Axes.Left.CalcYPosValue(TChart1.Axes.Left.MaxYValue)
Dim Height As Integer = TChart1.Axes.Left.CalcYPosValue(TChart1.Axes.Left.MinYValue) - TChart1.Axes.Left.CalcYPosValue(TChart1.Axes.Left.MaxYValue)
Dim Width As Integer = Points1.CalcXPos(5) - X
Dim R As New Rectangle(X, Y, Width, Height)
TChart1.Zoom.Animated = True
TChart1.Zoom.AnimatedSteps = 100
TChart1.Zoom.ZoomRect(R)
Zoom events
Zooming in manually, or by code, will trigger the TChart.Zoomed event. Zooming out will trigger the TChart.UndoneZoom event.
Scroll
Scroll is enabled in all directions as default. Use the Scroll.Allow property to disable Scroll or limit Scroll to one direction. The easiest way to scroll by code is to use the Axis Scroll method:
[C#]
tChart1.Axes.Bottom.Scroll(3, false);
[VB.Net]
TChart1.Axes.Bottom.Scroll(3, False)
The value is the offset. 'False' refers to whether or not TeeChart will allow scrolling beyond Series value limits.
Another way to control scroll is to define Axis maximum and minumum to scroll by code:
[C#]
private void Form1_Load(object sender, System.EventArgs e) {
int range = Convert.ToInt32(bar1.XValues.Maximum - bar1.XValues.Minimum / 2);
bar1.FillSampleValues(20);
tChart1.Panning.Allow = ScrollModes.None;
hScrollBar1.Value = range;
hScrollBar1.Minimum = range - 50;
hScrollBar1.Maximum = range + 50;
}
private void hScrollBar1_Scroll(object sender, System.Windows.Forms.ScrollEventArgs e) {
tChart1.Axes.Bottom.Automatic = false;
tChart1.Axes.Bottom.Minimum = e.NewValue;
tChart1.Axes.Bottom.Maximum = e.NewValue + bar1.Count;
}
[VB.Net]
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
Dim Range As Integer = Bar1.XValues.Maximum - Bar1.XValues.Minimum / 2
Bar1.FillSampleValues(20)
TChart1.Panning.Allow = Steema.TeeChart.ScrollModes.None
HScrollBar1.Value = Range
HScrollBar1.Minimum = Range - 50
HScrollBar1.Maximum = Range + 50
End Sub
Private Sub HScrollBar1_Scroll(ByVal sender As Object, ByVal e As System.Windows.Forms.ScrollEventArgs) Handles HScrollBar1.Scroll
TChart1.Axes.Bottom.Automatic = False
TChart1.Axes.Bottom.Minimum = e.NewValue
TChart1.Axes.Bottom.Maximum = e.NewValue + Bar1.Count
End Sub

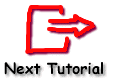